Klank
Althea ¤ A New World Developer
Greetings.
I made a drop and kill tracker module together with the public exp analyzer module found here.
Module does not use opcode, but by network messages.
Im still learning modules and programming in general, so if you find flaws or bad coding, please comment this thread, so that others and me can correct it.
Killer Tracker / Exp Tracker / Drop Tracker
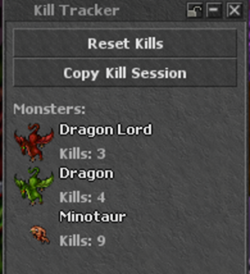
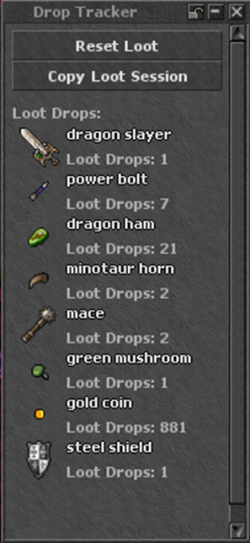
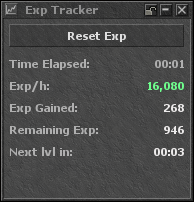
Analyzer Menu:
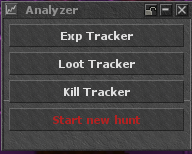
So what do you need to do?
Server-side Code
First of all, you need commit from the official TFS branch : Kill tracking (#3860) · otland/forgottenserver@ba72ce6 (https://github.com/otland/forgottenserver/commit/ba72ce6787668974b2f0b9db24747d4ac1693507)
And in addition, you need to add the item name to the network:
// msg:addString(item:getName())
As you might have noticed, there is a “true” in addItem. If the code works without true for you, you don’t need to add it. But if you need to, add this in luascript.cpp.
(This caused a lot of bugs for me in client, adding this to the additem method, at least solved the issue for me.)
Client side Code
Since we are not using opcodes, we need to edit the protocolgameparse.cpp. In OTCV8, the parsing for kill tracker is already reserved.
Replace this:
With this:
in localplayer.h, below ‘void setBlessings(int blessings);’ add:
In localplayer.cpp, at end of file, add:
That’s about it. Feel free to improve or add more features to the module.
I made a drop and kill tracker module together with the public exp analyzer module found here.
Module does not use opcode, but by network messages.
Im still learning modules and programming in general, so if you find flaws or bad coding, please comment this thread, so that others and me can correct it.
- Copy Kill or Drop session to Clipboard:
- Start new Hunt Button (opens all trackers)
- Reset singular trackers (ex Reset kills, resets kill tracker only)
- Trackers needs to be visible to track.
- Ctrl+H to open Window
- Tracks Number of killed creatures and items since the respective tracker was opened(resets on death or logout)
- Exp Session
Killer Tracker / Exp Tracker / Drop Tracker
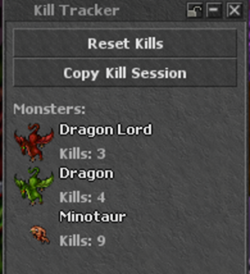
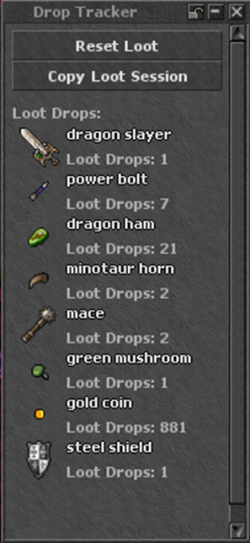
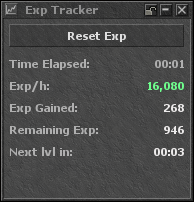
Analyzer Menu:
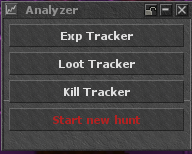
So what do you need to do?
Server-side Code
First of all, you need commit from the official TFS branch : Kill tracking (#3860) · otland/forgottenserver@ba72ce6 (https://github.com/otland/forgottenserver/commit/ba72ce6787668974b2f0b9db24747d4ac1693507)
And in addition, you need to add the item name to the network:
// msg:addString(item:getName())
Lua:
function Player.updateKillTracker(self, monster, corpse)
local monsterType = monster:getType()
if not monsterType then
return false
end
local msg = NetworkMessage()
msg:addByte(0xD1)
msg:addString(monster:getName())
local monsterOutfit = monsterType:getOutfit()
msg:addU16(monsterOutfit.lookType or 19)
msg:addByte(monsterOutfit.lookHead)
msg:addByte(monsterOutfit.lookBody)
msg:addByte(monsterOutfit.lookLegs)
msg:addByte(monsterOutfit.lookFeet)
msg:addByte(monsterOutfit.lookAddons)
local corpseSize = corpse:getSize()
msg:addByte(corpseSize)
for index = corpseSize - 1, 0, -1 do
local item = corpse:getItem(
index)
msg:addItem(item,true)
msg:addString(item:getName()) --- ADD THIS
end
local party = self:getParty()
if party then
local members = party:getMembers()
members[#members + 1] = party:getLeader()
for _, member in ipairs(members) do
msg:sendToPlayer(member)
end
else
msg:sendToPlayer(self)
end
msg:delete()
return true
end
(This caused a lot of bugs for me in client, adding this to the additem method, at least solved the issue for me.)
Code:
int LuaScriptInterface::luaNetworkMessageAddItem(lua_State* L)
{
// networkMessage:addItem(item, false/true)
Item* item = getUserdata<Item>(L, 2);
if (!item) {
reportErrorFunc(L, getErrorDesc(LUA_ERROR_ITEM_NOT_FOUND));
lua_pushnil(L);
return 1;
}
NetworkMessage* message = getUserdata<NetworkMessage>(L, 1);
bool description = getBoolean(L, 3, false);
if (message) {
message->addItem(item, description);
pushBoolean(L, true);
} else {
lua_pushnil(L);
}
return 1;
}
Client side Code
Since we are not using opcodes, we need to edit the protocolgameparse.cpp. In OTCV8, the parsing for kill tracker is already reserved.
Replace this:
Code:
void ProtocolGame::parseKillTracker(const InputMessagePtr& msg)
{
msg->getString();
msg->getU16();
msg->getU8();
msg->getU8();
msg->getU8();
msg->getU8();
msg->getU8();
int corpseSize = msg->getU8(); // corpse size
for (int i = 0; i < corpseSize; i++) {
getItem(msg); // corpse item
}
}
With this:
Code:
void ProtocolGame::parseKillTracker(const InputMessagePtr& msg)
{
std::string name = msg->getString(); //Monster Name
uint16_t lookType = msg->getU16(); //Monster Looktype
uint8_t lookHead = msg->getU8(); // Monster Head
uint8_t lookBody = msg->getU8(); // Monster Body
uint8_t lookLegs = msg->getU8(); // Monster Legs
uint8_t lookFeet = msg->getU8(); // Monster Feet
uint8_t addons = msg->getU8(); //Monster Addons
uint8_t corpseSize = msg->getU8(); // corpse size
std::vector<std::tuple<std::string, ItemPtr>> items;
for (int i = 0; i < corpseSize; i++) {
ItemPtr item = getItem(msg);
std::string itemName = msg->getString();
items.push_back(std::make_tuple(itemName, item));
}
m_localPlayer->updateKillTracker(name, lookType, lookHead, lookBody, lookLegs, lookFeet, addons, corpseSize, items);
}
In localplayer.h, below ‘void setBlessings(int blessings);’
void updateKillTracker(std::string name, uint16_t lookType, uint8_t lookHead, uint8_t lookBody, uint8_t lookLegs, uint8_t lookFeet, uint8_t addons, uint8_t corpseSize, const std::vector<std::tuple<std::string, ItemPtr>> items);
in localplayer.h, below ‘void setBlessings(int blessings);’ add:
Code:
void updateKillTracker(std::string name, uint16_t lookType, uint8_t lookHead, uint8_t lookBody, uint8_t lookLegs, uint8_t lookFeet, uint8_t addons, uint8_t corpseSize, const std::vector<std::tuple<std::string, ItemPtr>> items);
In localplayer.cpp, at end of file, add:
Code:
void LocalPlayer::updateKillTracker(std::string name, uint16_t lookType, uint8_t lookHead, uint8_t lookBody, uint8_t lookLegs, uint8_t lookFeet, uint8_t addons, uint8_t corpseSize, const std::vector<std::tuple<std::string, ItemPtr>> items)
{
callLuaField("onUpdateKillTracker", name, lookType, lookHead, lookBody, lookLegs, lookFeet, addons,corpseSize, items);
}
That’s about it. Feel free to improve or add more features to the module.
Attachments
-
game_huntanalyzer.rar6.2 KB · Views: 50 · VirusTotal